A bit of explanation here.
Firstly, this example program uses my device MAC addresses. If anyone else wants to run it, they will need to use their own MAC addresses.
Secondly, I hate trying to keep track of two programs and what version of which program is in which device. Another worry is that a program is loaded into the wrong device. So to get things working here, I load the same program into each device and it tests the device’s MAC address in order to act a device 1or device 2.
Thinking of a rainbow: red=1, orange=2, … each device changes color to show its device number, if its the only device turned on.
If both devices are active, then each sends random numbers to the other and the number is used to switch to another rainbow colour.
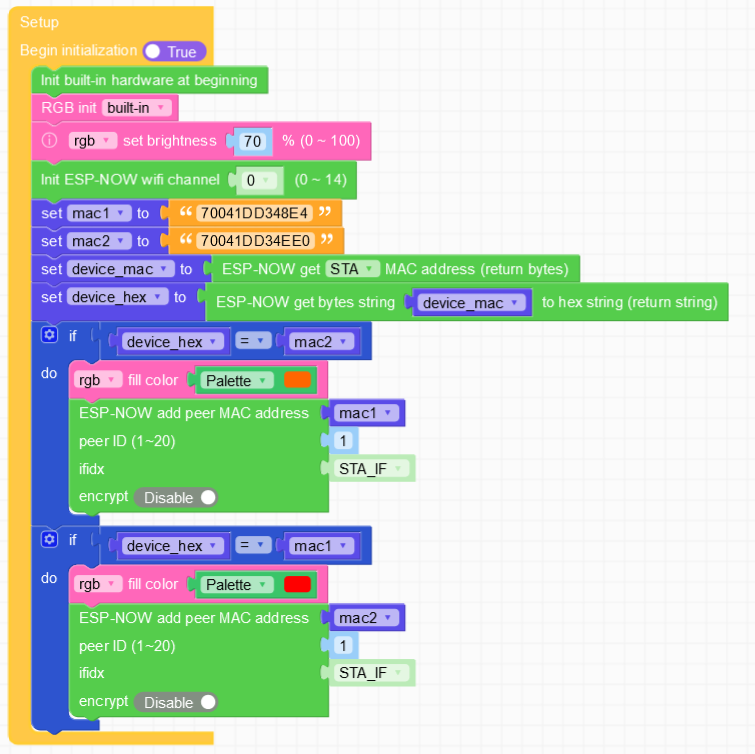
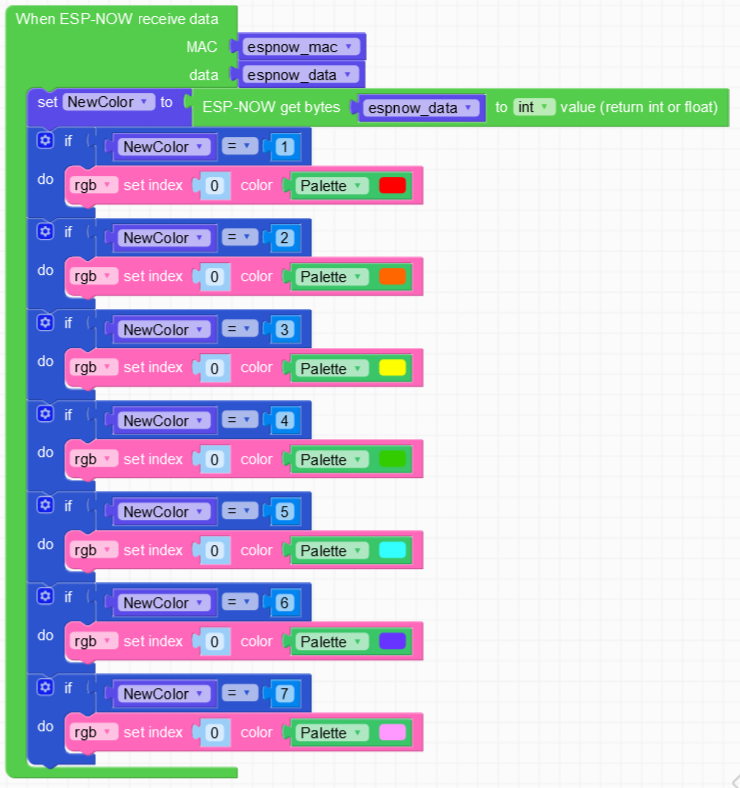
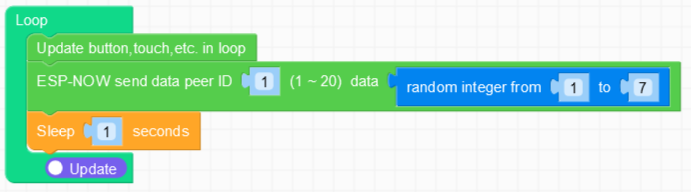
import os, sys, io
import M5
from M5 import *
from m5espnow import M5ESPNow
from hardware import *
import time
espnow_0 = None
rgb = None
import random
device_hex = None
espnow_mac = None
espnow_data = None
NewColor = None
mac1 = None
mac2 = None
device_mac = None
def espnow_recv_callback(espnow_obj):
global espnow_0, rgb, device_hex, espnow_mac, espnow_data, NewColor, mac1, mac2, device_mac
espnow_mac, espnow_data = espnow_obj.recv_data()
NewColor = espnow_0._bytes_to(espnow_data, 0)
if NewColor == 1:
rgb.set_color(0, 0xff0000)
if NewColor == 2:
rgb.set_color(0, 0xff6600)
if NewColor == 3:
rgb.set_color(0, 0xffff00)
if NewColor == 4:
rgb.set_color(0, 0x33cc00)
if NewColor == 5:
rgb.set_color(0, 0x33ffff)
if NewColor == 6:
rgb.set_color(0, 0x6633ff)
if NewColor == 7:
rgb.set_color(0, 0xff99ff)
def setup():
global espnow_0, rgb, device_hex, espnow_mac, espnow_data, NewColor, mac1, mac2, device_mac
M5.begin()
rgb = RGB()
rgb.set_brightness(70)
espnow_0 = M5ESPNow(0)
espnow_0.set_irq_callback(espnow_recv_callback)
mac1 = '70041DD348E4'
mac2 = '70041DD34EE0'
device_mac = espnow_0.get_mac(0)
device_hex = espnow_0._bytes_to_hex_str(device_mac)
if device_hex == mac2:
rgb.fill_color(0xff6600)
espnow_0.set_add_peer(mac1, 1, 0, False)
if device_hex == mac1:
rgb.fill_color(0xff0000)
espnow_0.set_add_peer(mac2, 1, 0, False)
def loop():
global espnow_0, rgb, device_hex, espnow_mac, espnow_data, NewColor, mac1, mac2, device_mac
M5.update()
espnow_0.send_data(1, random.randint(1, 7))
time.sleep(1)
if __name__ == '__main__':
try:
setup()
while True:
loop()
except (Exception, KeyboardInterrupt) as e:
try:
from utility import print_error_msg
print_error_msg(e)
except ImportError:
print("please update to latest firmware")